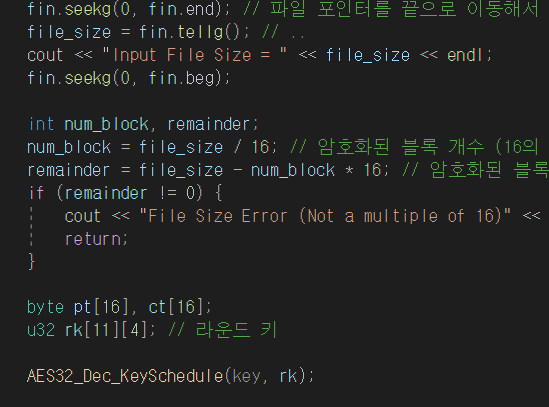
[C++] 블록암호 구현 - 파일 암/복호화 (AES_ECB)
oolongeya
·2021. 12. 12. 17:22
프로젝트에 위와 같은 파일들을 추가하여 AES 암복호화를 사용한다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
|
#include <iostream>
#include <fstream>
#include "AES32.h"
using namespace std;
typedef unsigned char byte;
// 0x80 패딩
void padding(byte in[], int in_length, byte out[16]) {
byte pad_byte = 0x80;
for (int i = 0; i < in_length; i++) {
out[i] = in[i];
}
out[in_length] = 0x80;
for (int i = in_length + 1; i < 16; i++) {
out[i] = 0x00;
}
}
// 패딩 제외 바이트 구하기
int pt_length(byte padded[16]) {
int position80;
position80 = 15;
for (int i = 15; i > 0; i--) {
if (padded[i] != 0x00) break;
position80--;
}
if (padded[position80] != 0x80) {
cout << "Padding error: 0x80 not found." << endl;
// (1) 0x00이 끝나는 지점에 0x80이 없을 때
// (2) 0x00이 계속 나올 때
return -1; // (1) or (2)
}
else {
return position80;
}
}
void AES_Enc_ECB(const char* pPT, byte key[16], const char* pCT) {
ifstream fin;
ofstream fout;
char ch;
fin.open(pPT, ios::binary);
if (fin.fail()) {
cout << "Input file open error!" << endl;
return;
}
fout.open(pCT, ios::binary);
if (fout.fail()) {
cout << "Output file open error!" << endl;
return;
}
// 파일 크기 확인
int file_size;
fin.seekg(0, fin.end); // 파일 포인터를 끝으로 이동해서 계산 하는 방식
file_size = fin.tellg(); // ..
cout << "Input File Size = " << file_size << endl;
fin.seekg(0, fin.beg);
int num_block, remainder;
num_block = file_size / 16 + 1; // 패딩 후 출력되는 블록 개수
remainder = file_size - (num_block - 1) * 16;
byte pt[16], ct[16];
u32 rk[11][4]; // 라운드 키
AES32_Enc_KeySchedule(key, rk);
for (int i = 0; i < num_block - 1; i++) {
fin.read((char*)pt, 16);
AES32_Encrypt(pt, rk, ct); // 암호화
fout.write((char*)ct, 16);
}
// 마지막 블록 => 패딩 처리 => 암호화
byte pt_pad[16];
for (int i = 0; i < remainder; i++) {
fin.read(&ch, 1);
pt[i] = ch;
}
padding(pt, remainder, pt_pad); // 패딩
AES32_Encrypt(pt_pad, rk, ct); // 암호화
fout.write((char*)ct, 16);
fin.close();
fout.close();
}
void AES_Dec_ECB(const char* pCT, byte key[16], const char* pDecPT) {
ifstream fin;
ofstream fout;
char ch;
fin.open(pCT, ios::binary);
if (fin.fail()) {
cout << "Input file open error!" << endl;
return;
}
fout.open(pDecPT, ios::binary);
if (fout.fail()) {
cout << "Output file open error!" << endl;
return;
}
// 파일 크기 확인
int file_size;
fin.seekg(0, fin.end); // 파일 포인터를 끝으로 이동해서 계산 하는 방식
file_size = fin.tellg(); // ..
cout << "Input File Size = " << file_size << endl;
fin.seekg(0, fin.beg);
int num_block, remainder;
num_block = file_size / 16; // 암호화된 블록 개수 (16의 배수 블록)
remainder = file_size - num_block * 16; // 암호화된 블록은 평문 블록보다 길 수 있음
if (remainder != 0) {
cout << "File Size Error (Not a multiple of 16)" << endl;
return;
}
byte pt[16], ct[16];
u32 rk[11][4]; // 라운드 키
AES32_Dec_KeySchedule(key, rk);
for (int i = 0; i < num_block - 1; i++) {
fin.read((char*)ct, 16);
AES32_EqDecrypt(ct, rk, pt); // 복호화
fout.write((char*)pt, 16);
}
fin.read((char*)ct, 16); // 마지막 블록
AES32_EqDecrypt(ct, rk, pt);
int last_pt_len; // 마지막 블록의 실제 데이터 길이 (0~15)
last_pt_len = pt_length(pt);
for (int i = 0; i < last_pt_len; i++) {
ch = pt[i];
fout.write(&ch, 1);
pt[i] = ch;
}
fin.close();
fout.close();
}
void File_ECB_test() {
const char* pPT = "PT.bin";
const char* pCT = "CT.bin";
const char* pDecPT = "DecPT.bin";
byte key[16] = { 0, };
for (int i = 0; i < 16; i++) {
key[i] = i;
}
cout << "AES ECB Encrypt" << endl;
AES_Enc_ECB(pPT, key, pCT);
cout << "AES ECB Encrypt" << endl;
AES_Dec_ECB(pCT, key, pDecPT);
}
int main()
{
File_ECB_test();
}
|
cs |
반응형
'암호학 (Cryptology)' 카테고리의 다른 글
[C++] 블록암호 구현 - 구현 검증 (AES_AVS) (0) | 2021.12.12 |
---|---|
[python] 차분 공격 (Differential Attack) 키 공격 코드 (0) | 2021.11.26 |